How to Create a Fitness App in Android Studio in 2025
Learn how to create a fitness app in Android Studio, get some example fitness app Android Studio source code, and learn how to make a fitness app even quicker.
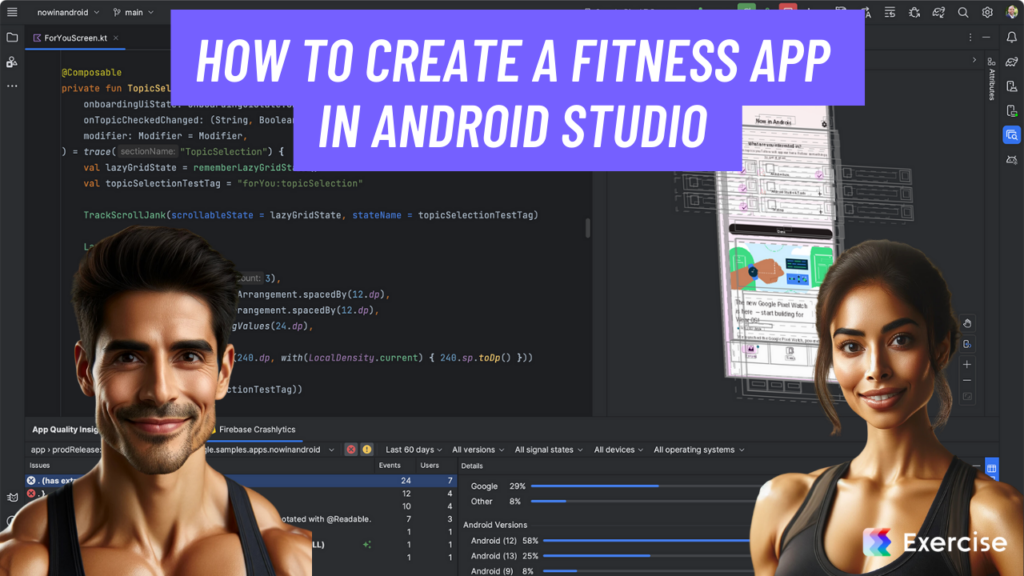
Creating a fitness app in Android Studio is a technically intensive process that involves several steps, from setting up the development environment to coding and testing. However, while building a custom fitness app from scratch provides control over every aspect, using a platform like Exercise.com can significantly reduce development time and complexity. Here’s a detailed guide on how to build a fitness app in Android Studio, along with insights on why opting for Exercise.com might be a more efficient choice.
Creating a workout tracking app typically involves several key steps, each requiring careful planning and execution. The process can be significantly streamlined by using a platform like Exercise.com, which not only provides the necessary tools but also ensures that the app meets the specific needs of fitness professionals. This approach with the best white label fitness app builder software from Exercise.com avoids the high costs and long timelines associated with hiring even the best fitness development agencies and the extensive time investment needed to build an app from the ground up.
Publish your custom branded fitness apps so you can offer a premium workout logging experience to your community.
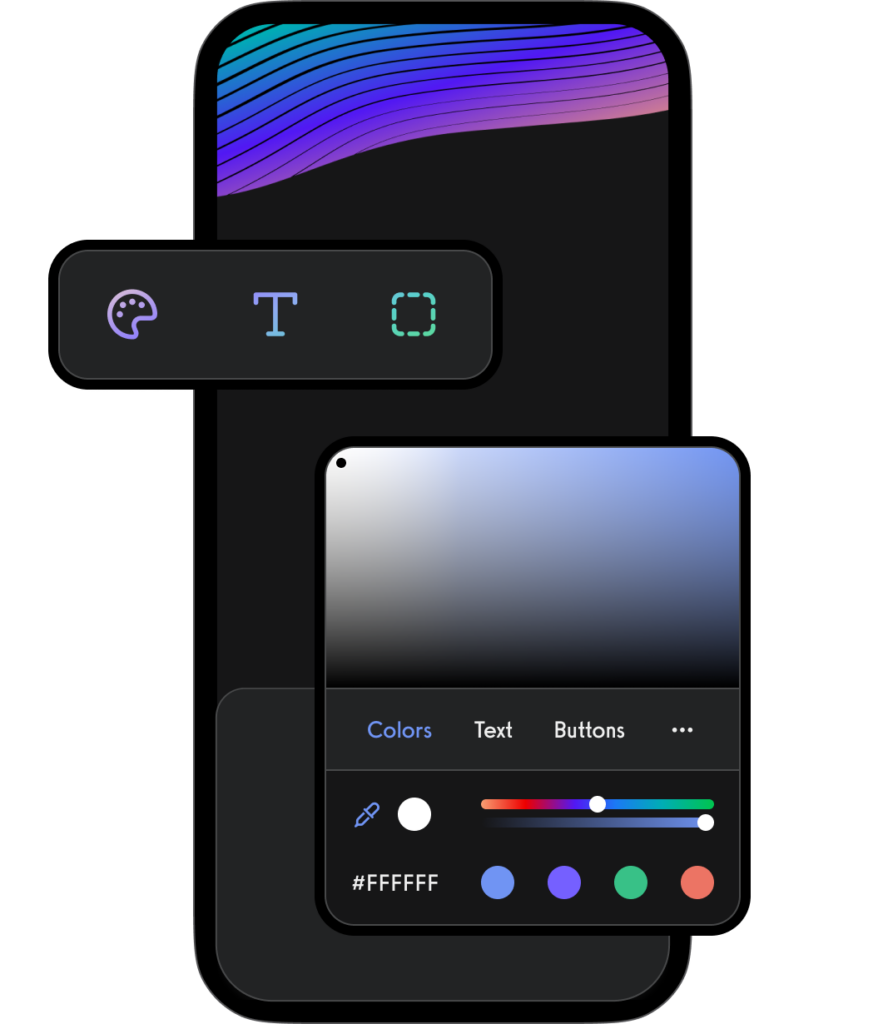
Read on for a step-by-step guide on how to make a fitness app in Android Studio and then decide if you want to leverage Exercise.com to build your fitness app with no coding required. See why the best gym software, the best personal training software, and the best fitness software for fitness influencers is all found on the Exercise.com platform.
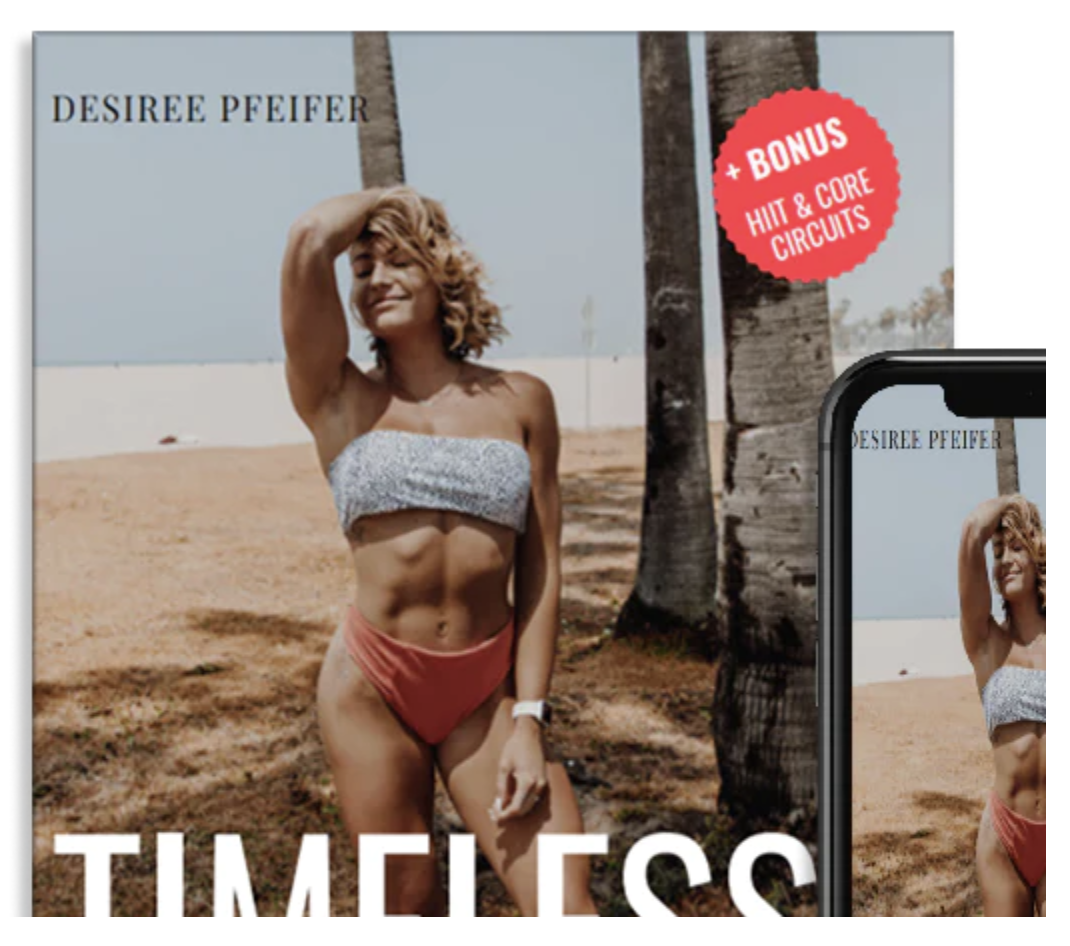
Step #1: Set Up Android Studio Environment
- Installation: Download and install Android Studio from the official Android website. Ensure your system meets the software’s requirements.
- Project Setup: Start a new Android Studio project. Choose a template that suits a basic fitness app, like the “Basic Activity” template to get a default layout and navigation.
- Dependencies: Add necessary dependencies in your
build.gradle
file for components you’ll need, such as Google Fit API for activity tracking and Retrofit for network requests.
Why Exercise.com is Better: Exercise.com already has a robust environment set up with all necessary integrations, eliminating the need for complex fitness app code environment setup and fitness app source code maintenance.
Step #2: Design the User Interface
- Layout Editor: Use Android Studioās Layout Editor to design the UI. Create screens for user login, activity tracking, diet logging, and more.
- Custom Components: Design custom views if the default components donāt meet your needs. For example, create a custom view to display a userās daily step count progress.
- Responsive Design: Ensure your app is responsive and looks good on all device sizes by using ConstraintLayout and properly managing resource directories.
Why Exercise.com is Better: With Exercise.com, you can leverage professionally designed templates that are optimized for user engagement and conversion, ensuring a high-quality user experience without the need for deep design expertise.
Step #3: Implement Core Functionalities
- Activity Tracking: Integrate with the Google Fit API to access and display data like steps, calories burned, and active minutes.
- Nutrition Tracking: If your app includes dietary tracking, integrate a third-party API or develop a custom solution to log and analyze food intake.
- User Authentication: Implement Firebase Authentication to handle user registration and login securely.
Why Exercise.com is Better: Exercise.com provides built-in functionalities for activity tracking, nutrition, and user management, all secured and maintained by industry experts, saving you the trouble of coding these features from scratch.
Read More: Types of Fitness Apps
Step #4: Data Management
- Local Database: Use Room or SQLite for local data storage to save user data such as workout logs and preferences.
- Cloud Sync: Implement cloud storage solutions like Firebase Cloud Firestore to sync and store user data across devices.
Why Exercise.com is Better: Exercise.com includes secure data storage and synchronization across devices, ensuring user data is always accessible and up-to-date without any additional coding required.
Step #5: Testing and Debugging
- Emulator Testing: Test your app extensively in Android Studioās emulator. Simulate different conditions and use cases to check app behavior.
- Physical Device Testing: Test the app on actual devices to ensure it performs well in real-world conditions.
- Debugging: Use Android Studioās debugging tools to identify and fix bugs. Check logs and monitor app performance to optimize.
Why Exercise.com is Better: Exercise.comās platform has been rigorously tested across many scenarios and devices, ensuring reliability and reducing the time you spend on debugging and testing.
Step #6: Launch and Maintenance
- Google Play Submission: Prepare your app for submission. Create a compelling app store listing, gather all necessary metadata, and submit your app to Google Play.
- Updates and Iterations: Regularly update the app based on user feedback and evolving requirements.
Why Exercise.com is Better: With Exercise.com, you donāt need to worry about the app store submission process or ongoing updates. The platform handles everything, allowing you to focus on growing your fitness business.
While building a fitness app in Android Studio gives you complete control and customization, it requires significant time, technical skill, and maintenance. Choosing Exercise.com offers a comprehensive, ready-to-deploy solution that lets you launch quickly with less effort and more support. Ready to start building your fitness app the easy way? Book a demo with Exercise.com today and see how you can bring your fitness app to market faster and more efficiently.
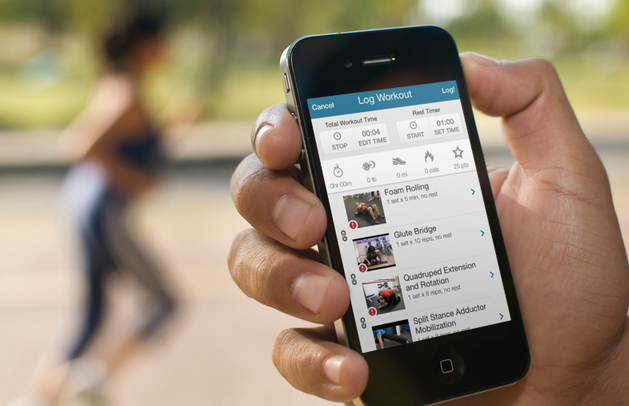
Example Fitness App Android Studio Source Code
Creating a simple fitness app in Android Studio involves several steps, from setting up the basic user interface to integrating functionalities like activity tracking. Below, I’ll provide a basic example of Android Studio source code for a simple fitness app. This app will include basic features like a step counter and a simple user interface for displaying the step count.
Step 1: Set Up Your Android Studio Project
- Start a New Project: Open Android Studio and start a new project with an Empty Activity.
- Name Your Project: For example, “SimpleFitnessApp”.
- Set Up Your Environment: Choose API level 21 or higher to support most devices.
Step 2: Update Your build.gradle
(Module: app)
Include the necessary dependencies for sensors as we’ll use the device’s hardware to count steps.
dependencies {
implementation 'androidx.appcompat:appcompat:1.2.0'
implementation 'androidx.constraintlayout:constraintlayout:2.0.4'
implementation 'com.google.android.gms:play-services-fitness:20.0.0'
implementation 'com.google.android.gms:play-services-auth:19.0.0'
testImplementation 'junit:junit:4.+'
androidTestImplementation 'androidx.test.ext:junit:1.1.2'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.3.0'
}
Step 3: Add Permissions to AndroidManifest.xml
You need to request permission to access the user’s activity data.
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.simplefitnessapp">
<uses-permission android:name="android.permission.ACTIVITY_RECOGNITION"/>
<application
...
>
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
</activity>
...
</application>
</manifest>
Step 4: Design the Layout
Create a simple layout in res/layout/activity_main.xml
with a TextView to display the step count.
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textViewSteps"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Steps: 0"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Step 5: Implement Step Counter in MainActivity.java
Use the Sensor API to access the step counter sensor and update the TextView.
package com.example.simplefitnessapp;
import android.hardware.Sensor;
import android.hardware.SensorEvent;
import android.hardware.SensorEventListener;
import android.hardware.SensorManager;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity implements SensorEventListener {
private TextView textViewSteps;
private SensorManager sensorManager;
private Sensor stepSensor;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textViewSteps = findViewById(R.id.textViewSteps);
sensorManager = (SensorManager) getSystemService(SENSOR_SERVICE);
stepSensor = sensorManager.getDefaultSensor(Sensor.TYPE_STEP_COUNTER);
if (stepSensor == null) {
textViewSteps.setText("No Step Counter Sensor!");
}
}
@Override
protected void onResume() {
super.onResume();
sensorManager.registerListener(this, stepSensor, SensorManager.SENSOR_DELAY_UI);
}
@Override
protected void onPause() {
super.onPause();
sensorManager.unregisterListener(this);
}
@Override
public void onSensorChanged(SensorEvent event) {
if (event.sensor.getType() == Sensor.TYPE_STEP_COUNTER) {
textViewSteps.setText("Steps: " + (int) event.values[0]);
}
}
@Override
public void onAccuracyChanged(Sensor sensor, int accuracy) {
// Required method but can be left empty
}
}
Getting Started
This basic fitness app in Android Studio demonstrates a simple way to track steps using the device’s hardware. However, building a full-featured fitness app involves much more complexity, including robust backend services, additional health tracking features, user authentication, and data security.
Using a platform like Exercise.com offers a comprehensive solution that bypasses these complexities, providing all the tools needed to launch and manage a fitness app efficiently. Exercise.com streamlines app development, allowing you to focus on delivering value to your users with professional support, built-in functionalities, and a secure environment. Ready to take your fitness app to the next level? Book a demo with Exercise.com today.
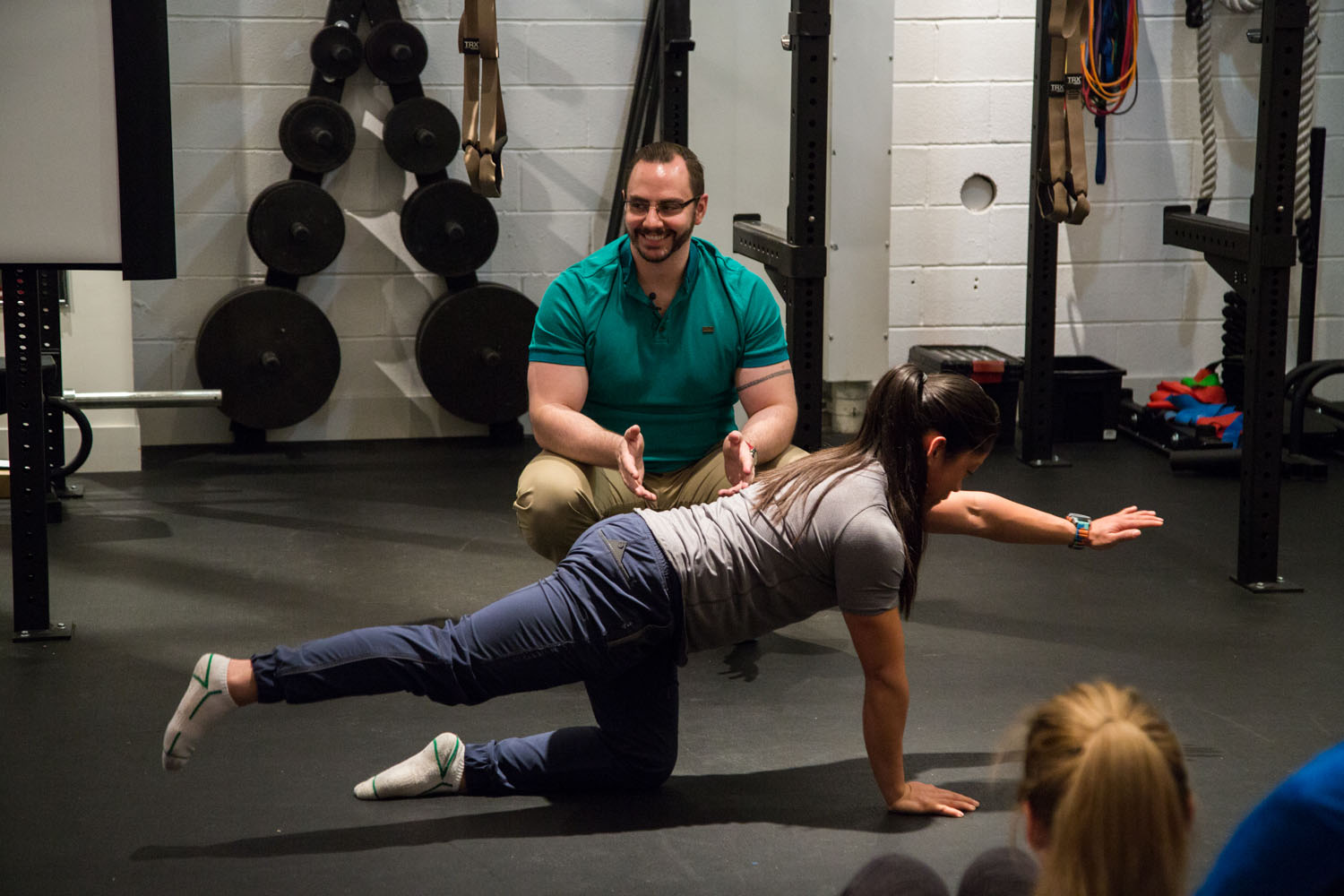
Core Android Studio Fitness App Functionalities and Integrations
Creating a fitness app in Android Studio can be an educational and challenging project, especially for those looking to learn more about app development, integration, and dependencies. Here’s a comprehensive list of various types of integrations and dependencies that might be required for a fitness app project:
Firebase Integration
- Purpose: To manage user authentication, database storage, analytics, and push notifications.
- Details: Firebase provides a backend-as-a-service solution that offers a wide range of services including real-time databases, user authentication (Google, Facebook login), and cloud storage, which are essential for a dynamic fitness app.
Google Fit API
- Purpose: To access health and activity data collected from various fitness devices and sensors.
- Details: Integrating Google Fit allows your app to retrieve user activity data like steps, calorie expenditure, and heart rate, essential for tracking fitness progress.
AdMob Integration
- Purpose: To incorporate ads into your app as a revenue stream.
- Details: AdMob by Google is one of the worldās largest mobile ad platforms that can help monetize your app by displaying engaging ads that are relevant to your users.
Maps and Location Services
- Purpose: To track and record outdoor activities such as running, cycling, or walking routes.
- Details: Integrating Google Maps or similar services allows you to add location tracking, enhancing functionality for apps that monitor outdoor fitness activities.
Payment Gateway Integration
- Purpose: To facilitate in-app purchases and subscriptions.
- Details: Integrations with payment systems like Stripe, PayPal, or Google Pay are crucial for processing transactions securely, enabling users to purchase subscriptions or premium content.
Wearable Device Integration
- Purpose: To sync data from various wearable devices.
- Details: Integrations with Apple HealthKit or similar services allow the app to access data from a multitude of devices, providing a holistic view of the userās health and activity.
Social Media Integration
- Purpose: To enable users to share their fitness achievements on social media.
- Details: Integrating social media APIs like Facebook or Instagram can boost user engagement and provide free marketing as users share their progress.
Analytics Integration
- Purpose: To track user behavior and app performance.
- Details: Tools like Google Analytics or Firebase Analytics help you understand how users interact with your app, which can inform future improvements.
Third-Party Fitness APIs
- Purpose: To enrich the app with various fitness and health data services.
- Details: APIs like Nutritionix for dietary tracking or OpenWeatherMap for incorporating weather information can enhance the appās functionality.
Machine Learning Integration
- Purpose: To provide personalized fitness and health recommendations.
- Details: Leveraging ML Kit or TensorFlow Lite can enable features like activity recognition, personalized workout and diet plans based on user behavior and preferences.
Using a Professional Solution Like Exercise.com
While building a fitness app from scratch can be an enriching learning experience, it requires handling complex integrations and continuous maintenance. For fitness professionals aiming to launch a robust app quickly and efficiently, using a professional solution like Exercise.com is advisable. Exercise.com provides a comprehensive platform with all the necessary features pre-built and integrated, from user management and workout creation to payment processing and analytics. This not only speeds up the time to market but also ensures a high-quality, reliable product that is scalable and secure.
By choosing Exercise.com, fitness professionals can focus on what they do best ā training clients and growing their fitness business ā without the technical headaches of app development. Ready to launch your fitness app with a trusted, all-in-one platform? Book a demo with Exercise.com today and start your journey with a powerful tool tailored to your business needs.
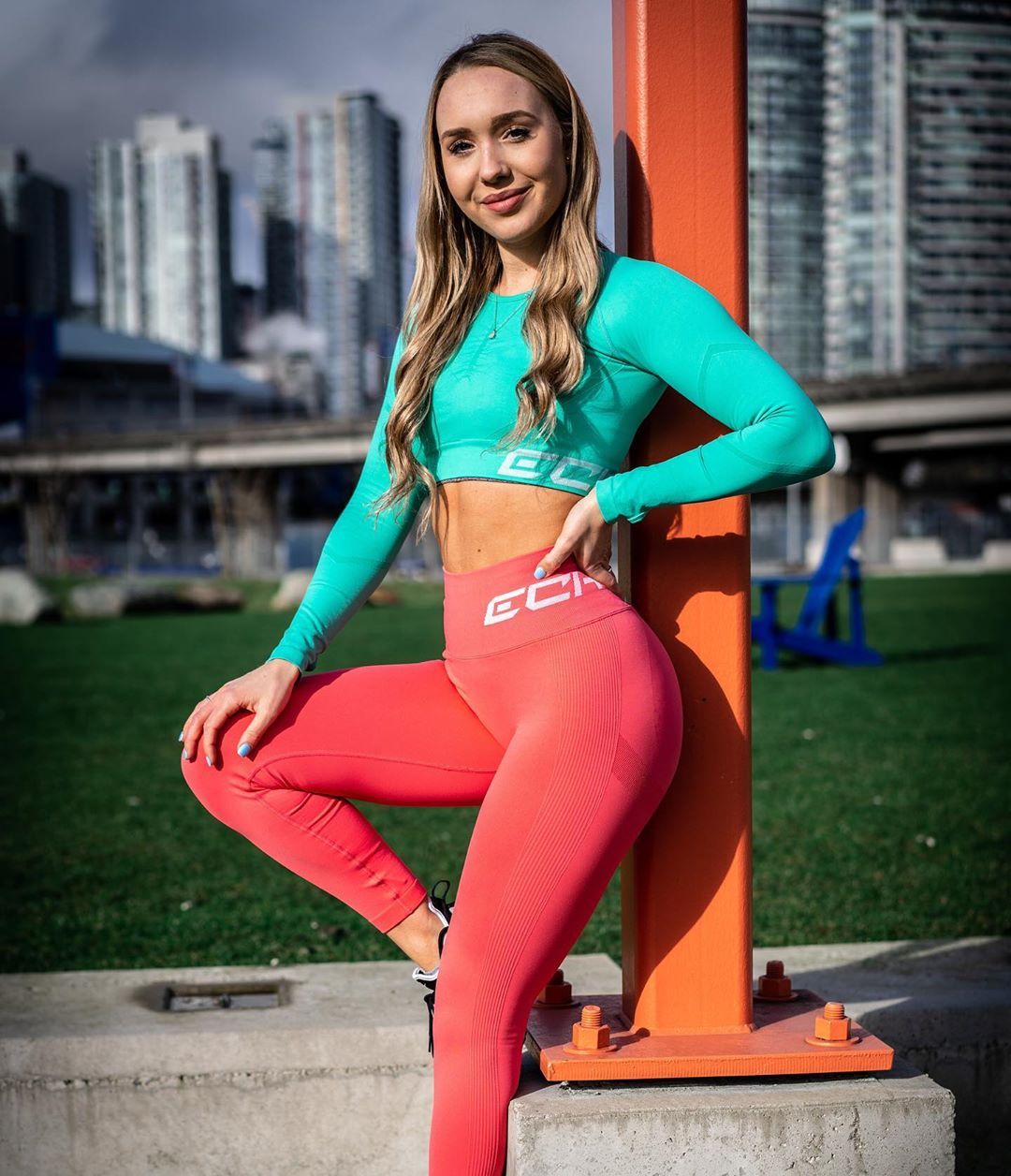
How do I make an Android fitness app?
To make an Android fitness app, you’ll typically start by defining your app’s core functionalities, such as activity tracking, nutrition logging, or social networking features. The development process involves designing a user-friendly interface and programming the app using Android Studio, which is the integrated development environment recommended for Android app development. You’ll need to have a good grasp of programming languages like Java or Kotlin. If you’re not a developer, hiring a software development team is a practical approach. For fitness professionals looking to launch an app without extensive technical expertise, platforms like Exercise.com offer a comprehensive solution. Exercise.com allows you to create custom-branded fitness apps that integrate seamlessly with your business model, providing features like workout creation, client and class management, and e-commerce without needing to code. This not only simplifies the app development process but also ensures that the app is perfectly aligned with your business goals.
Read More:
- How to Make a Fitness App
- Best Fitness App Development Companies
- How to Make a Weight Loss App
- How to Make a Fitness Influencer App
Can I make my own fitness app?
Yes, you can make your own fitness app by either learning how to use app development tools like Android Studio or outsourcing the development to professionals. To succeed, you should define clear objectives for what you want your app to achieve, such as delivering personalized workouts, tracking user progress, or providing nutritional guidance. For those without coding skills, using a platform like Exercise.com is highly advantageous. Exercise.com offers the tools to create a custom-branded fitness app tailored to your business needs, making it easier to engage with clients and manage different aspects of your fitness business efficiently.
Can I build an app with Android Studio?
Yes, you can build an app using Android Studio, which is the official Integrated Development Environment (IDE) for Android app development. It provides all the tools necessary to code, test, and deploy your application. You will need to be familiar with Java or Kotlin, as these are the primary languages supported by Android Studio. For beginners, there are numerous tutorials and resources available online to help learn how to use Android Studio effectively.
How do I create a fitness app in MIT App Inventor?
Creating a fitness app with MIT App Inventor is easy and accessible. First, set up your environment by creating an account on the MIT App Inventor website and installing the MIT AI2 Companion app on your Android device. Start a new project by clicking “Projects” and “Start new project,” then design your user interface by dragging components like buttons and textboxes from the Palette to the Viewer. Customize these components in the Properties pane. Next, switch to the Blocks editor to program your appās behavior using visual blocks, creating event handlers and control flows. Test your app by connecting your device through the AI Companion app, scanning the QR code for live testing. Save your project, build the app, and distribute it via QR code or .apk file. While MIT App Inventor is great for beginners, Exercise.com offers a comprehensive platform for creating and managing professional-grade workout plans. With features like personal training program design templates, exercise program design template, and detailed tracking with personal training tracking sheets, Exercise.com is the superior choice for fitness professionals looking to streamline their operations and deliver top-notch services.
Read More: How do I create a fitness app in MIT App Inventor?
How do you make a fitness tracker app?
To make a fitness tracker app, start by deciding on the key features, such as step counting, activity logging, and calorie tracking. Develop the app using a suitable platform like Android Studio or a cross-platform solution to cater to a broader audience. For accurate data collection and enhanced functionalities, integrate sensors and APIs that can track physical activities and health metrics. Ensure the app has a user-friendly interface and strong data privacy measures. For those seeking a simpler route, platforms like Exercise.com offer development tools and services that can help launch a fitness tracker app with comprehensive features without the need for deep technical expertise.
How much does it cost to build a fitness app?
The cost to build a fitness app can vary widely based on complexity, features, and whether you hire freelance developers or a development agency. A basic app might start around $10,000, while a more feature-rich app could cost $100,000 or more. Factors affecting the price include the appās design, backend development, and ongoing maintenance. Utilizing a platform like Exercise.com can be a cost-effective solution as it provides a comprehensive suite of tools tailored for fitness businesses, which reduces the need for extensive custom development.
How much does it cost to develop a fitness app?
Developing a fitness app typically involves similar costs to building any mobile app, which can range from $10,000 for a simple app to over $100,000 for a high-end app with multiple features like video streaming, community platforms, and advanced analytics. The final cost will depend on the app’s complexity, the design specifics, the number of platforms it supports, and whether you use in-house developers or outsource. Platforms like Exercise.com offer a more budget-friendly approach by providing customizable app templates that reduce both development time and cost.
How do I make a fitness app without coding?
To make a fitness app without coding, consider using app builders that offer drag-and-drop interfaces and customizable templates, such as Exercise.com. These platforms provide the necessary infrastructure and tools to create and manage a fitness app, including handling workouts, memberships, and online payments. This approach allows you to focus on the content and business aspects of your app without getting bogged down by technical details.
How do I make a free fitness app?
Creating a free fitness app generally involves using free app development platforms that offer basic functionalities at no cost. Keep in mind that while the app may be free to develop, maintaining and updating the app may incur costs. Alternatively, consider monetizing your free app through in-app advertisements, premium upgrades, or affiliate marketing to cover your operational expenses.
How long does it take to build a fitness app in Android Studio?
Building a fitness app in Android Studio can take anywhere from several months to over a year, depending on the appās complexity and the development team’s experience. The process includes planning, design, development, testing, and deployment phases. Using a platform like Exercise.com can significantly speed up this process by providing customizable app templates specifically designed for fitness businesses.
Can I build a fitness app on Android Studio without coding?
Building a fitness app on Android Studio generally requires coding knowledge in Java or Kotlin. However, there are several tools and plugins available that can simplify some aspects of the app development process, such as designing the user interface, without extensive coding. For a completely coding-free experience, consider using a platform like Exercise.com.
Do you need Android Studio to make Android apps?
While Android Studio is the official IDE recommended by Google for Android app development, it is not the only tool available. Other environments and frameworks like Xamarin, React Native, and Flutter allow for Android app development, often with the added benefit of cross-platform compatibility. However, for native Android development, Android Studio is typically the best choice due to its comprehensive toolset and integration with Google’s services.
How much does it cost to develop a fitness wearable app?
Developing a fitness wearable app can be more expensive than a standard mobile app due to the need for integrating with specific hardware and managing real-time data. Costs can range from $20,000 to over $100,000 depending on the app’s functionality and the complexity of the wearable technology. Ensuring compatibility with multiple types of devices also adds to the development time and cost.
Does Android have a fitness app?
Yes, Android devices can access numerous fitness apps through the Google Play Store. These range from simple pedometers to comprehensive fitness tracking suites that monitor a wide array of physical activities and health metrics.
How do I make my Android fitness app wearable?
To make your Android fitness app compatible with wearables, you’ll need to develop a Wear OS version of your app or ensure your existing app is compatible with Google’s Wear OS APIs. This involves adapting the appās interface for smaller screens and integrating with the wearable’s sensors to track fitness activities effectively.
How do I make money with fitness?
Making money in the fitness industry can be achieved through several avenues:
- Membership Fees: Charging for access to exclusive content, classes, or facilities.
- Personal Training: Offering personalized coaching and training plans.
- Selling Products: Marketing fitness-related products such as equipment, supplements, or apparel.
- Online Programs: Creating and selling digital fitness programs or subscriptions.
- Fitness Apps: Developing a fitness app and monetizing it through subscriptions, ads, or in-app purchases.
Platforms like Exercise.com can facilitate these revenue streams by providing tools to manage and grow your fitness business effectively online.
Read More: How do I make money with fitness?
How do I start a fitness business?
Starting a fitness business involves several key steps:
- Market Research: Understand your target market and the competition.
- Business Plan: Outline your business model, services, pricing, and marketing strategy.
- Certifications: Obtain any necessary fitness certifications and business licenses.
- Location and Equipment: Choose a location for your fitness center and acquire the necessary equipment.
- Online Presence: Develop a website and use social media to promote your services.
- Management Software: Implement software like Exercise.com to manage clients, classes, and payments efficiently.
By following these steps and utilizing reliable software, you can establish a successful fitness business.
Read More: How to Start a Fitness Business
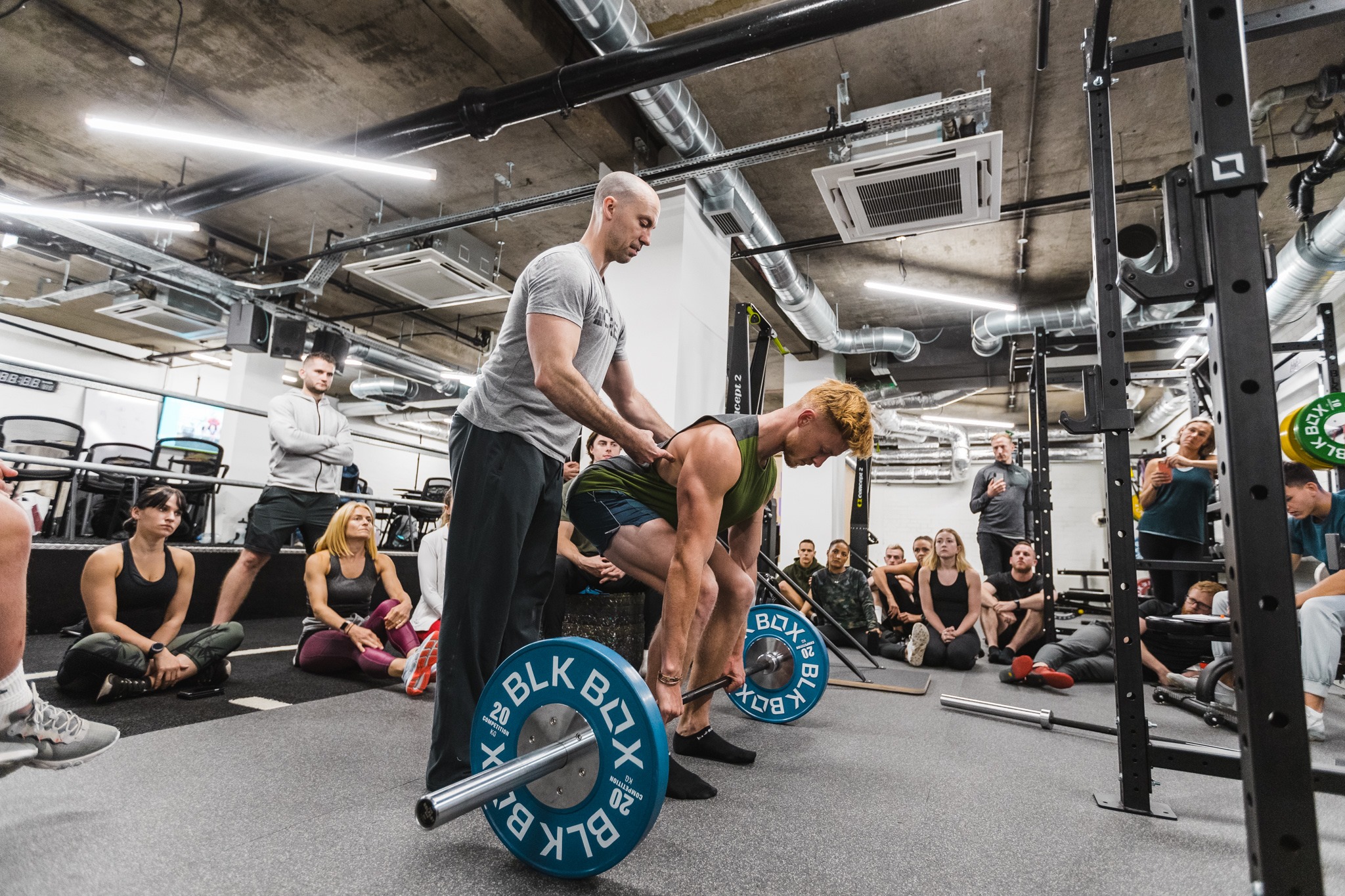